- 设置相关
Lambda 匿名函数
有时候槽函数代码辑逻辑非常简单,可以直接用下面的
Lambda匿名函数
处理信号,简捷明了.需c++11
支持,不支持自身递归调用.1
2
3
4
5
6
7QComboBox *cb = new QComboBox(this);
QObject::connect(cb,&QComboBox::currentTextChanged,[=](QString txt){
[...]
baseform->changeJsonValue(btn,uname,
baseform->mWindow->mItemMap.key(txt));
[...]
});函数内部的匿名函数
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
void ActionList::onCustomContextMenu(const QPoint &pos)
{
[...]
auto sawp_lambda_func = [this](int src,int dst) {
QVariantList oldvals;
for(int i = 0 ; i < mTable->columnCount();i++)
{
QWidget *w = mTable->cellWidget(src,i);
if(!QString::compare(w->metaObject()->className(),"QComboBox"))
{
oldvals.append(((QComboBox*)w)->currentText());
}else{
oldvals.append(((QLineEdit*)w)->text());
}
QObject::disconnect(w);
mTable->removeCellWidget(src,i);
w->deleteLater();
}
mTable->removeRow(src);
[...]
};
QAction up(QIcon(":/icon/icons/act_up.png") ,
QString("上移一行"),this);
QObject::connect(&up,
&QAction::triggered,[=](){
sawp_lambda_func(line,line-1);
});
[...]
}
模拟事件
一些
GUI
的操作,需要一些事件来完成,下面模拟一个鼠标Release
的事件1
2
3
4
5[...]
QMouseEvent *event = new QMouseEvent(QMouseEvent::MouseButtonRelease,QCursor::pos(),
Qt::LeftButton,Qt::LeftButton,Qt::NoModifier);
QApplication::postEvent(this,event);
[...]把事件转换成对其它控件的事件
1 | void NewGrid::wheelEvent(QWheelEvent *event) |
元对像,动态属性
Meta-Object System 的基本功能
Meta Object System
的设计基于以下几个基础设施:QObject
类- 作为每一个需要利用元对象系统的类的基类
QOBJECT
宏- 定义在每一个类的私有数据段,用来启用元对象功能,比如,动态属性,信号和槽
- 元对象编译器
moc (the Meta Object Complier)
- moc分析
C++
源文件,如果它发现在一个头文件(header file)中包含Q_OBJECT
宏定义,然后动态的生成另外一个C++
源文件,这个新的源文件包含Q_OBJECT
实现代码,这个新的C++
源文件也会被编译、链接到这个类的二进制代码中去,因为它也是这个类的完整的一部分.通常,这个新的C++
源文件会在以前的C++
源文件名前面加上moc
作为新文件的文件名.其具体过程如下图所示:
- moc分析
- 除了提供在对象间进行通讯的机制外,元对象系统还包含以下几种功能:
QObject::metaObject()
方法- 它获得与一个类相关联的
meta-object
-QMetaObject::className()
方法 - 在运行期间返回一个对象的类名,它不需要本地
C++
编译器的RTTI(run-time type information)
支持
- 它获得与一个类相关联的
QObject::inherits()
方法- 它用来判断生成一个对象类是不是从一个特定的类继承出来,当然,这必须是在
QObject
类的直接或者间接派生类当中
- 它用来判断生成一个对象类是不是从一个特定的类继承出来,当然,这必须是在
QObject::tr()
andQObject::trUtf8()
- 这两个方法为软件的国际化翻译字符串
QObject::setProperty()
andQObject::property()
- 这两个方法根据属性名动态的设置和获取属性值,读写属性
1 | QWidget *w = new QWidget() |
布署安装
对于开发QT程序来说,最好布署方法就是编译成静态执行文件,只是文件大一点.如果是动态编译就会有一些动态库链接路径的问题.比较特别的它要信赖
platforms
这个文件路经.可以通过 **QCoreApplication::addLibraryPath(“C:/WINDOWS/System32”);**来指定.参考文档,从样本模版里复制一份出来做相应的修改.
一个安装包的目录结构如下:
1 | D:\QtDev\QtInstaller\netmon-root>tree /F |
- package.xml
1 |
|
- config.xml
1 |
|
- isntallscript.qs
1 |
|
- Page.ui
1 | <?xml version="1.0" encoding="UTF-8"?> |
- 最后这里用一行脚本来生成一键安装包文件.
1 | binarycreator --offline-only -c D:\QtDev\QtInstaller\netmon-root\config\config.xml -p D:\QtDev\QtInstaller\netmon-root\packages "Z:/upload/appname-测试版%DATE%-%TIME::=_%.exe" |
调试变量
Qt plugin
版本不兼容的问题,有时Qt
莫名闪退,需设置export QT_DEBUG_PLUGINS=1
,方可以看下面的加载.1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29Found metadata in lib /fullpath/plugins/sqldrivers/libqsqlite.so, metadata=
{
"IID": "org.qt-project.Qt.QSqlDriverFactoryInterface",
"MetaData": {
"Keys": [
"QSQLITE"
]
},
"archreq": 0,
"className": "QSQLiteDriverPlugin",
"debug": false,
"version": 331264
}
Got keys from plugin meta data ("QSQLITE")
QFactoryLoader::QFactoryLoader() looking at "/fullpath/plugins/sqldrivers/libsqlitecipher.
Found metadata in lib /fullpath/plugins/sqldrivers/libsqlitecipher.so, metadata=
{
"IID": "org.qt-project.Qt.QSqlDriverFactoryInterface",
"MetaData": {
"Keys": [
"SQLITECIPHER"
]
},
"className": "SqliteCipherDriverPlugin",
"debug": false,
"version": 330499
}使用
export QT_FATAL_WARNINGS=1
,可以让程序的在警告的位置崩溃退出,并打印调用stack frame
.Could not initialize GLX
错误,尝试设置export QT_XCB_GL_INTEGRATION=none
是可以处理.export USE_WOLFRAM_LD_LIBRARY_PATH=1
1 | QXcbIntegration: Cannot create platform OpenGL context, neither GLX nor EGL are enabled |
关于跨平台的一些全局宏定义如:
Q_OS_WIN,Q_OS_MAC,...
可以查询参考https://doc.qt.io/qt-5/qtglobal.html对于工程内的模块,不要加上
CONFIG += debug_and_release
或者CONFIG += build_all
配置,除非是要把它做动态库发布出去的文件,否则这只会增加编译时间,编译了一些必要的对像.
QtCreator
调试设置
QtCreator
的调试插件Using Debugging Helpers,可以参考它,对GDB
做一些调脚本.常规选项如,Debugger -> Locals & Expressions -> Display string legnth
,默认100
字节,可能对于调一些大的json
结构体会不够.
Visual Studio
上的开发
安装
- 通过下载Visual Studio Community安装,在安装时需要注意的是,除了安装相应的开发工具套件外,还应该安装
开发-扩展(Visual Studio extension develepoment)
,通过它可以安装一些高效开发插件,如: Qt Visual Studio Tools,C++ Debugger Visualizers for VS2019,支持Qt
调试查看对像内容.
导入Qt
工程
msvc
也是一个很复杂,很高效的开发工具,如果在windows上调试,还是为它最合适,比竟都是它自家的东西.所以可以通过使用qmake -tp vc -r <your project>.pro
的方式,把Qt
工程转换成msvc
工程,转换后的工程,可能需要少许的手动修改.把
cl.exe
所在位置加入到,系统Path
变量里去,用power shell
运行qmake -tp vc ../your-project.pro
.或者,通过
开始
菜单,找到Visual Studio 2019
目录,运行x86 Native Tools Command Prompt for VS 2019
, 切换到工程目录运行qmake -tp vc
.或者,进入
C:\Program Files (x86)\Microsoft Visual Studio\2019\Community\VC\Auxiliary\Build
,运行相应的环境Shell.1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20cd "C:\Program Files (x86)\Microsoft Visual Studio\2019\Community\VC\Auxiliary\Build"
PS C:\Program Files (x86)\Microsoft Visual Studio\2019\Community\VC\Auxiliary\Build> ls
目录: C:\Program Files (x86)\Microsoft Visual Studio\2019\Community\VC\Auxiliary\Build
Mode LastWriteTime Length Name
---- ------------- ------ ----
d----- 2021/5/11 9:26 14.16
-a---- 2021/5/11 9:20 13 Microsoft.VCRedistVersion.default.txt
-a---- 2021/5/11 9:20 393 Microsoft.VCToolsVersion.default.props
-a---- 2021/5/11 9:20 13 Microsoft.VCToolsVersion.default.txt
-a---- 2021/5/11 9:26 401 Microsoft.VCToolsVersion.v141.default.props
-a---- 2021/5/11 9:26 13 Microsoft.VCToolsVersion.v141.default.txt
-a---- 2021/5/11 9:20 401 Microsoft.VCToolsVersion.v142.default.props
-a---- 2021/5/11 9:20 13 Microsoft.VCToolsVersion.v142.default.txt
-a---- 2021/5/11 9:20 39 vcvars32.bat
-a---- 2021/5/11 9:20 39 vcvars64.bat
-a---- 2021/5/11 9:20 9859 vcvarsall.bat
-a---- 2021/5/11 9:20 43 vcvarsamd64_x86.bat
-a---- 2021/5/11 9:20 43 vcvarsx86_amd64.bat命令行编译QT,
Build QT Command Line for Window1
qmake -spec win32-msvc "CONFIG+=qtquickcompiler" ../src/youduqt.pro
为
UTF-8
类型添加BOM
标识,因为windows
下有些程序需要认它.1
2printf '\xEF\xBB\xBF' > report_new.csv
cat report >> report_new.csv或者这样
1
sed -i '1s/^/\xef\xbb\xbf/' file-encoded-with-utf8.txt
vs2019
奇怪错误,vs2019 C1075
, 这个问题在Linux
下编译正常,在windows
下出错,这是因为这个文件是在Linux
下创建,不是UTF8-BOM
,所以才出错.这是在不系统下切换开发环境才会碰到的.
调试设置
- IDE_Debug_Helpers
- Natvis
- 把一些
*.natvis
文件放入到C:\Program Files (x86)\Microsoft Visual Studio\2019\Community\Common7\Packages\Debugger\Visualizers
里.可以支持调试Qt
工程序时,查看对像内的内容,而不是一个指针值.
一些工程总结
- 工程拆分模块,尽量可以动/静态库的方式链接或者耦合,面向接口编程.
- 对于可以共用的模块功能,中间的类名,尽量以抽像方式命名,不要带有具体名称,尤其是产品名,公司名.如果要把它移到别的产品就会有点别扭.
- 对于一些相同功能的类,又没有共同继父类的,类之间尽量不要有全名匹配相同的函数名,当工程到了一规模时,搜索函数名时可以高效过滤找到.
- 对于大的工程,尽量不要滥用,如:
use namespace std;
, 对于规模比较大的工程,文件行数多时,在review
时无法直观知道它所属的命名空间,而因此碰到命名冲突的概率
也很高. - 成员变量必须要有一个前缀,如:
m_XXXXX
,方便有别于局部变量区分.
错误总结
Qt5
加载QSS
资源问题,这个是因为QSS
的语法或者配置错误所造成的,会使整个QSS
环境坏了.遇到过因为border-image: url(:/x_wnd/home_086@2x.png);
而出现下面错误.因为url
里的路径里含有@
字符,对于路径最好是用双引号(“”)包括起来.而一般的UI工程师切图导出的文件,就是带@
符号来区分尺寸的.但是发现在QT C++
里写obj->setButtonImage(":/main_wnd/new/02_003_01@2x.png");
是可以识别,是正常可行的.1
2
3
4
5Could not parse stylesheet of object 0x55dabc30bd60
Could not parse stylesheet of object 0x55dabc2e8220
Could not parse stylesheet of object 0x55dabc30bd60
Could not parse stylesheet of object 0x55dabc30bd60
Could not parse stylesheet of object 0x55dabc30bd60
QWebChannel使用
谢谢支持
- 微信二维码:
- 联系作者
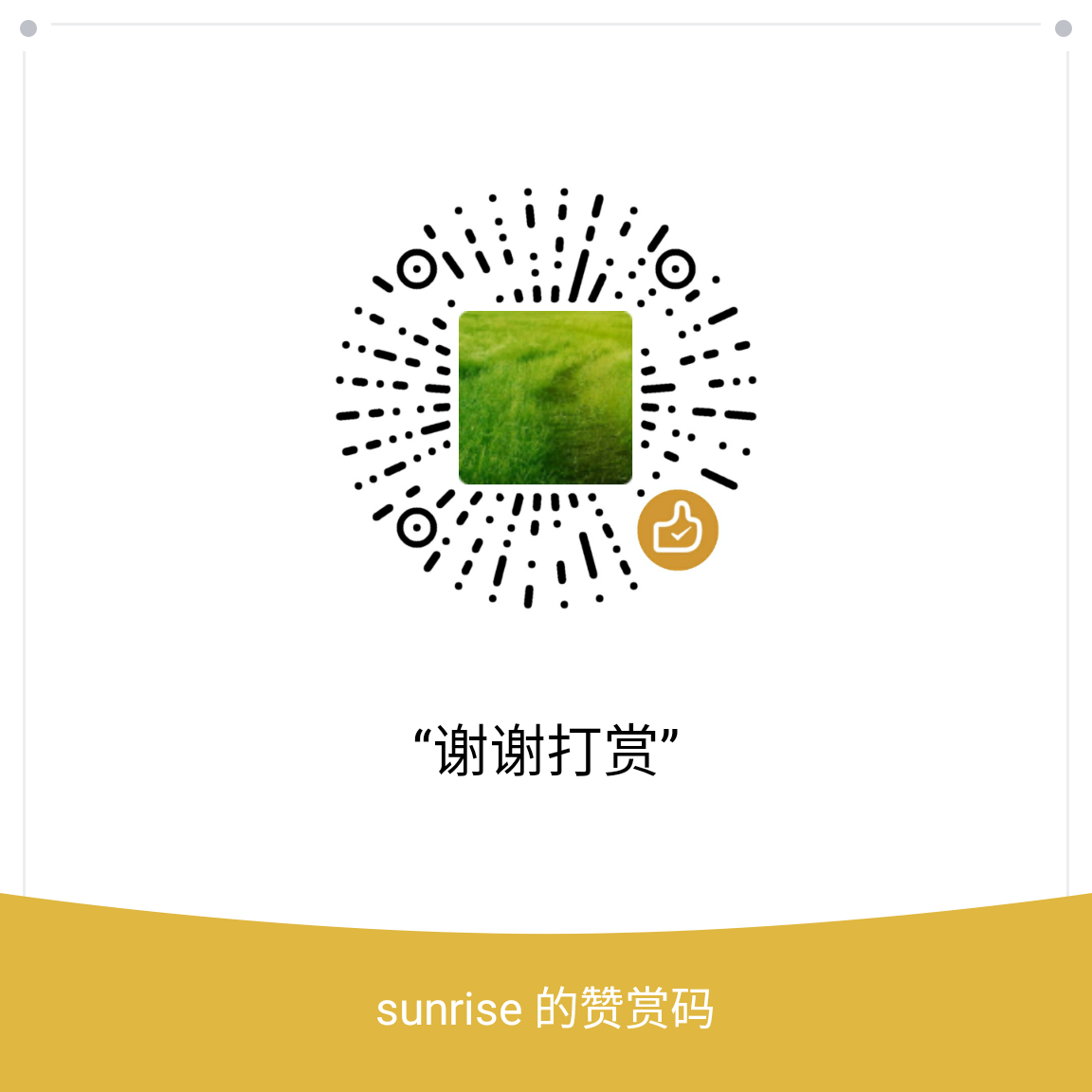